Chatbots are versatile applications that can be employed in various fields and industries to assist users, automate tasks, provide information, and engage in conversations. ChatterBot is a Python library that allows developers to create chatbots that can engage in text-based conversations. It uses a combination of machine learning techniques and predefined conversational datasets to simulate human-like interactions. ChatterBot is designed to facilitate easy development and integration of chatbots into various applications.
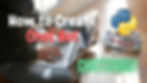
The objective of the project tutorial is to build a chatbot application using python. The chatbot can work in retrieval based and generative based. You can create a general chatbot with the existing corpus (or) create a custom data for the related domain for any business purpose.
You can watch the video-based tutorial with step by step explanation down below.
Install Modules
pip install chatterbot
pip install chatterbot_corpus
You have to install the chatterbot library and its associated corpus using pip.
Import Modules
from chatterbot import ChatBot
from chatterbot.trainers import ListTrainer, ChatterBotCorpusTrainer
chatterbot - used for creating chatbots that can engage in text-based conversations with users. It provides a framework for developing simple chatbot applications, primarily based on predefined conversational data and rule-based responses.
chatterbot.trainers - contains classes that are used to train chatbots with conversational data, allowing them to generate meaningful responses during interactions.
ChatterBotCorpusTrainer - This trainer uses pre-existing conversational datasets available within the ChatterBot library. It's a quick way to train the chatbot using a variety of language patterns and responses from the included datasets.
ListTrainer - The ListTrainer allows you to train the chatbot with custom lists of conversations. This can be useful when you want to provide specific conversation data. to your chatbot.
Initialize the Chatbot
First we will initialize the chatbot.
# initialize the chatbot
hrbot = ChatBot(name='HackersRealmBot', read_only=True, logic_adapters=['chatterbot.logic.BestMatch'])
[nltk_data] Downloading package averaged_perceptron_tagger to
[nltk_data] C:\Users\Aswin\AppData\Roaming\nltk_data...
[nltk_data] Package averaged_perceptron_tagger is already up-to-
[nltk_data] date!
[nltk_data] Downloading package punkt to
[nltk_data] C:\Users\Aswin\AppData\Roaming\nltk_data...
[nltk_data] Package punkt is already up-to-date!
[nltk_data] Downloading package stopwords to
[nltk_data] C:\Users\Aswin\AppData\Roaming\nltk_data...
[nltk_data] Package stopwords is already up-to-date!
You will initialize a chatbot named hrbot using the ChatterBot library with the BestMatch logic adapter. The BestMatch logic adapter is commonly used with ChatterBot to provide responses based on the closest matching input pattern.
name: This parameter specifies the name of the chatbot. In your case, it's set to 'HackersRealmBot'.
read_only: This parameter is set to True, which means that the chatbot will only provide responses and won't learn or update its knowledge during interactions.
logic_adapters: This parameter specifies the list of logic adapters to be used for generating responses.
By using the BestMatch logic adapter, your chatbot will attempt to match user input to patterns it has learned during training and provide the most relevant response based on those patterns.
Train and Test the Chatbot with Corpus
Next we will train the chatbot using the chatterbot corpus trainer.
corpus_trainer = ChatterBotCorpusTrainer(hrbot)
corpus_trainer.train('chatterbot.corpus.english')
Training ai.yml: [####################] 100%
Training botprofile.yml: [####################] 100%
Training computers.yml: [####################] 100%
Training conversations.yml: [####################] 100%
Training emotion.yml: [####################] 100%
Training food.yml: [####################] 100%
Training gossip.yml: [####################] 100%
Training greetings.yml: [####################] 100%
Training health.yml: [####################] 100%
Training history.yml: [####################] 100%
Training humor.yml: [####################] 100%
Training literature.yml: [####################] 100%
Training money.yml: [####################] 100%
Training movies.yml: [####################] 100%
Training politics.yml: [####################] 100%
Training psychology.yml: [####################] 100%
Training science.yml: [####################] 100%
Training sports.yml: [####################] 100%
Training trivia.yml: [####################] 100%
corpus_trainer = ChatterBotCorpusTrainer(hrbot): This line creates an instance of the ChatterBotCorpusTrainer and associates it with your chatbot (hrbot). This trainer will be used to train your chatbot on the corpus data.
corpus_trainer.train('chatterbot.corpus.english'): This line initiates the training process. The trainer will load the English language corpus that comes with ChatterBot and train your chatbot using the patterns and responses present in the corpus.
Next let us try to print the response from your chatbot when given the input "Hello."
print(hrbot.get_response('Hello'))
Hi
Next let us test the chatbot by giving continuous input.
while True:
input_text = input('You:')
if input_text == 'quit':
break
print('HR Bot:', hrbot.get_response(input_text))
You: hello
HR Bot: Hi
You: how are you?
HR Bot: I am on the Internet.
You: who are you
HR Bot: I am just an artificial intelligence.
You: quit
while True: loop ensures that the conversation continues indefinitely until you decide to quit.
input_text = input('You:'): This line prompts you to enter your message. The input message is stored in the input_text variable.
if input_text == 'quit': This condition checks if you've entered "quit." If you do, the loop breaks, and the conversation ends.
print('HR Bot:', hrbot.get_response(input_text)): If the input is not "quit," the chatbot generates a response using the hrbot.get_response(input_text) function and prints it as "HR Bot:" followed by the response.
Now you'll be able to have back-and-forth interactions with your chatbot. When you're done, you can type "quit" to exit the conversation.
Train and Test the Chatbot with Custom Input
Let us create a custom input list of questions and replies.
train_list = ['what is your name?', 'I am Hackers realm bot. How can I help you', 'I am HR Bot. Nice to meet you', 'which language you are using?', 'I am using python language']
Next let us train the chatbot with custom input.
list_trainer = ListTrainer(hrbot)
list_trainer.train(train_list)
list_trainer = ListTrainer(hrbot): This line creates an instance of the ListTrainer and associates it with your chatbot (hrbot). This trainer will be used to train your chatbot using the custom list of conversations you provide.
list_trainer.train(train_list): This line initiates the training process using the provided train_list. The train_list should be a list of strings representing conversations. Each string in the list should contain both the user's input and the chatbot's response, separated by a newline character (\n).
After training with the custom list of conversations, your chatbot (hrbot) should be able to respond to inputs similar to the ones you've provided in the train_list.
Next let us test the chatbot.
while True:
input_text = input('You: ')
if input_text == 'quit':
break
print('HR Bot:', hrbot.get_response(input_text))
You: hi
HR Bot: How are you doing?
You: i am fine
HR Bot: you are not here to
You: what is your name?
HR Bot: I am Hackers realm bot. How can I help you
You: what is you name
HR Bot: The character of Lt. Commander Data was written to come across as being software-like, so it is natural that there is a resemblance between us.
You: what is your name?
HR Bot: I am Hackers realm bot. How can I help you
You: which language you are using
HR Bot: I am using python language
You: quit
while True: loop ensures that the conversation continues indefinitely until you decide to quit.
input_text = input('You:'): This line prompts you to enter your message. The input message is stored in the input_text variable.
if input_text == 'quit': This condition checks if you've entered "quit." If you do, the loop breaks, and the conversation ends.
print('HR Bot:', hrbot.get_response(input_text)): If the input is not "quit," the chatbot generates a response using the hrbot.get_response(input_text) function and prints it as "HR Bot:" followed by the response.
You can see that when you have asked questions defined in the custom list then we have got the appropriate response from the custom list.
Final Thoughts
Define the purpose and goals of your chatbot. Determine whether you want a simple Q&A bot, a more complex conversational bot, or a specialized domain-specific bot.
Depending on your requirements, choose the appropriate library or framework. ChatterBot is a good starting point for simple chatbots, but for more advanced capabilities, consider Rasa, Dialogflow, or custom solutions using spaCy and machine learning models.
Test your chatbot thoroughly with various input scenarios. Collect feedback and refine the bot's responses based on user interactions.
In this tutorial we have seen how you can create a basic chat bot using python. However, creating a chatbot is an iterative process. Start with a basic version, gather insights, and gradually enhance its capabilities based on user needs and feedback. As you delve into the world of chatbots, you'll gain valuable experience in NLP, AI, and user-centered design.
Get the project notebook from here
Thanks for reading the article!!!
Check out more project videos from the YouTube channel Hackers Realm